Ray Tracer Project
I implemented a ray tracer with rigid body dynamics for my final CS488 project. Unfortunately I didn’t have time to complete collision detection for all primitives, and could only demonstrate colliding spheres.
Ray Tracer with Rigid Body Dynamics
Table of Contents
- Dynamic Objects
- Rigid Body Collisions
- Texture Mapping
- Bump Mapping
- Multi-threaded Rendering
- Intensity Threshold Optimization
- Antialiasing
- Additional Primitives
- Constructive Solid Geometry
- Rube Goldberg machine
Objective 1 & 2: Dynamic Objects
Example of two spheres colliding
Objective 3: Texture Mapping
The images show texture mapping for all primitives, a simple mesh (background) and a CSG object.
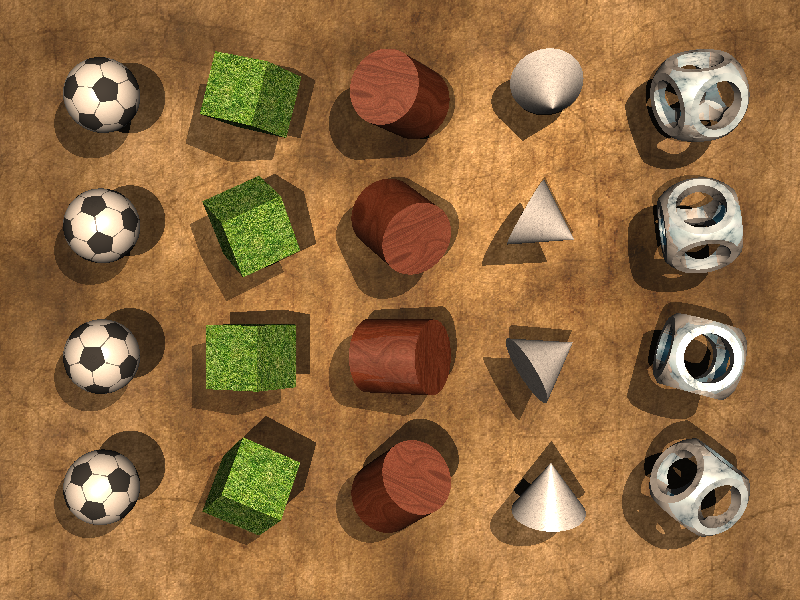
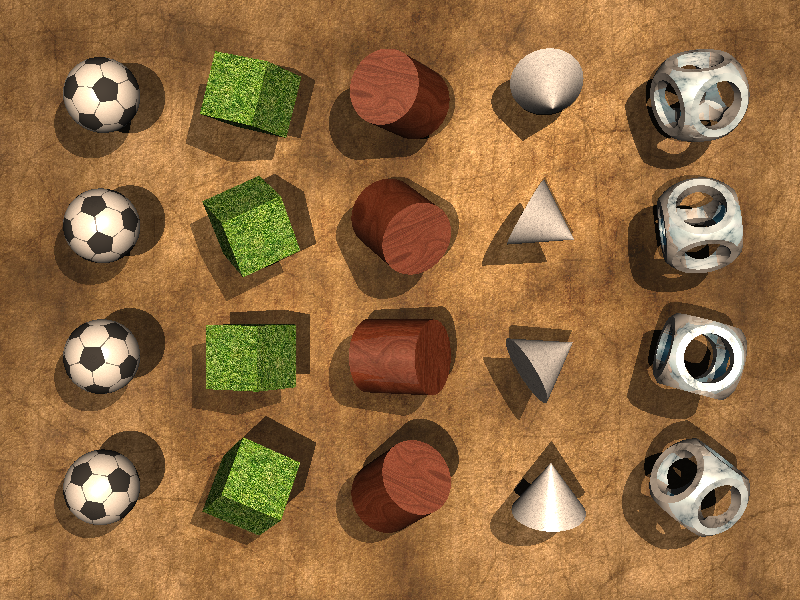
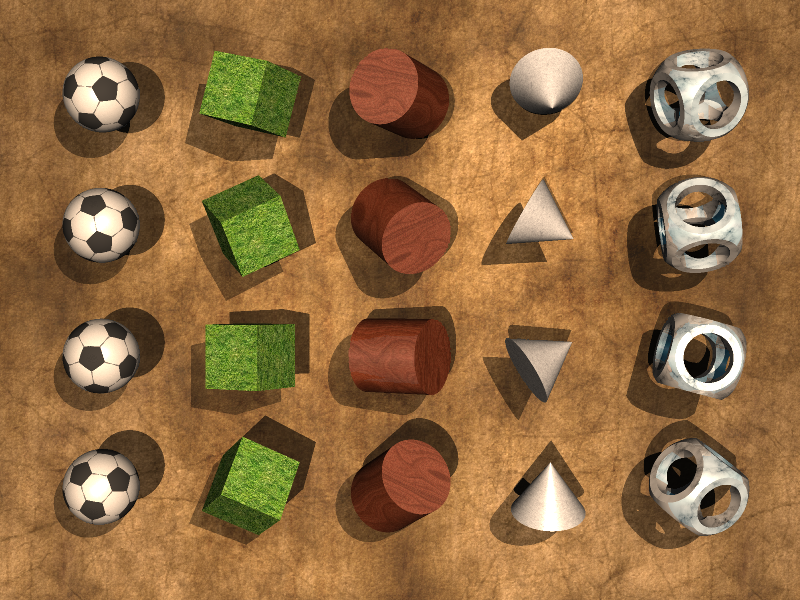
Objective 4: Bump Mapping
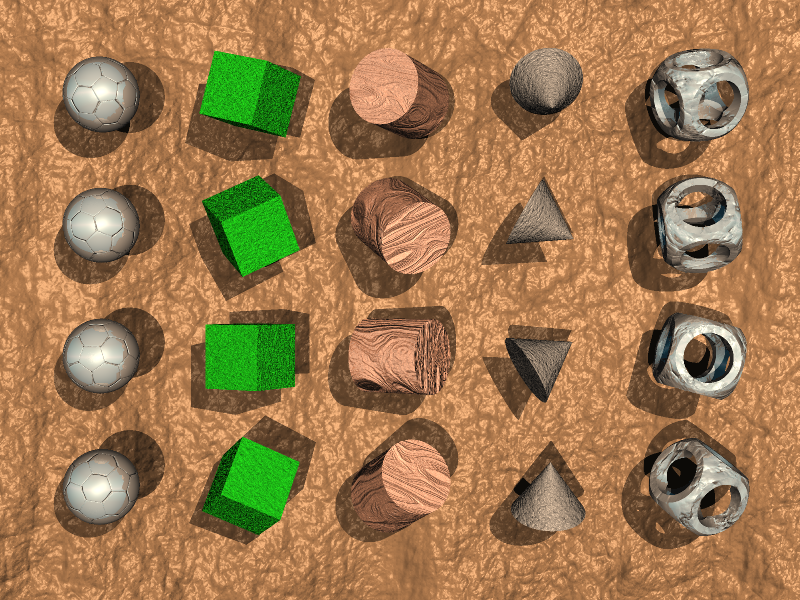
Objective 5: Multi-threaded Rendering
The final scene (see Objective 10), which has one frame, is rendered with a varying number of threads. All features of the ray tracer were enabled. The following renders were performed with the unix “time” command, on the following machine:
gl25.student.cs Asus based AMD quad core, 4gb ram, 2x500g hd, Asus ENGTX260GL
# of Threads | real | user | sys |
---|---|---|---|
n = 1 | 274.23s | 273.321s | 0.704s |
n = 5 | 108.91s | 269.028s | 0.669s |
n = 10 | 93.64s | 268.232s | 0.776s |
n = 20 | 81.41s | 270.123s | 0.732s |
n = 50 | 98.66s | 301.526s | 61.047s |
Thus running the ray tracer with 20 threads speeds up the run time by 3.37 times, or by 3 minutes and 12 seconds, which is a significant improvement.
Objective 6: Intensity Threshold Optimization
I used my final scene to demonstrate the gain of the intensity threshold optimization, which actually showed a significant increase in performance.
Antialiasing was disabled, the reflection depth was set at 5, and 4 threads was used. The threshold used was large value of 0.000001. The following renders were performed with the unix “time” command, on the following machine
gl25.student.cs Asus based AMD quad core, 4gb ram, 2x500g hd, Asus ENGTX260GL
Threshold | No Threshold | |
---|---|---|
real | 16.90s | 40.13s |
user | 39.094s | 131.640s |
sys | 0.568s | 0.608s |
Thus we have found a significant increase in performance (~ 2.5 times) by using the intensity threshold check. Note that without the check, 1752362 more shader computations were made.
Objective 7: Anti-aliasing
The given scene (slightly modified) from CS488 Assignment 4 with polyhedral cows is used to demonstrate antialiasing
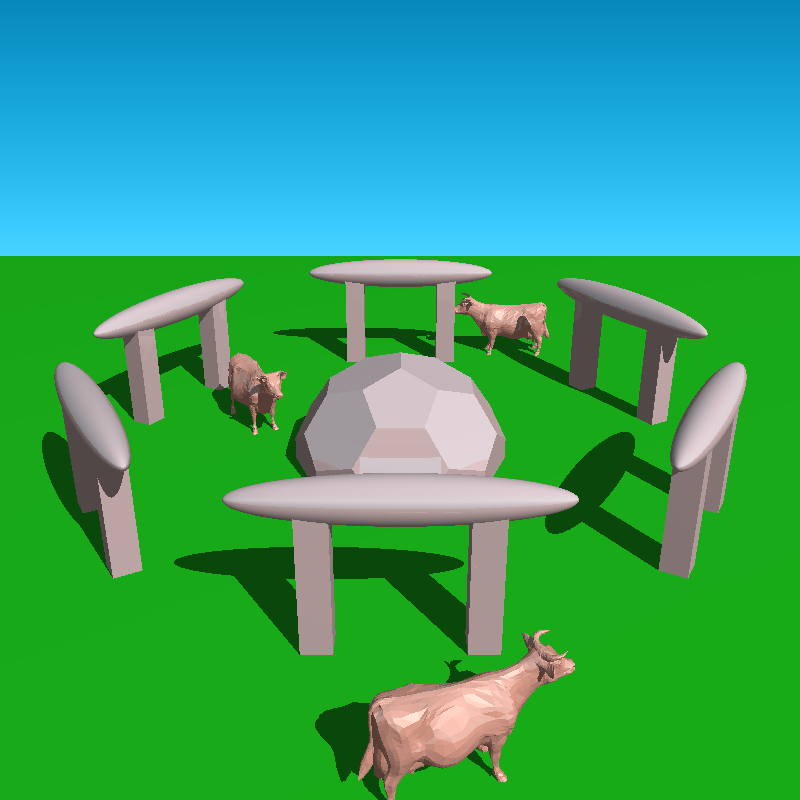
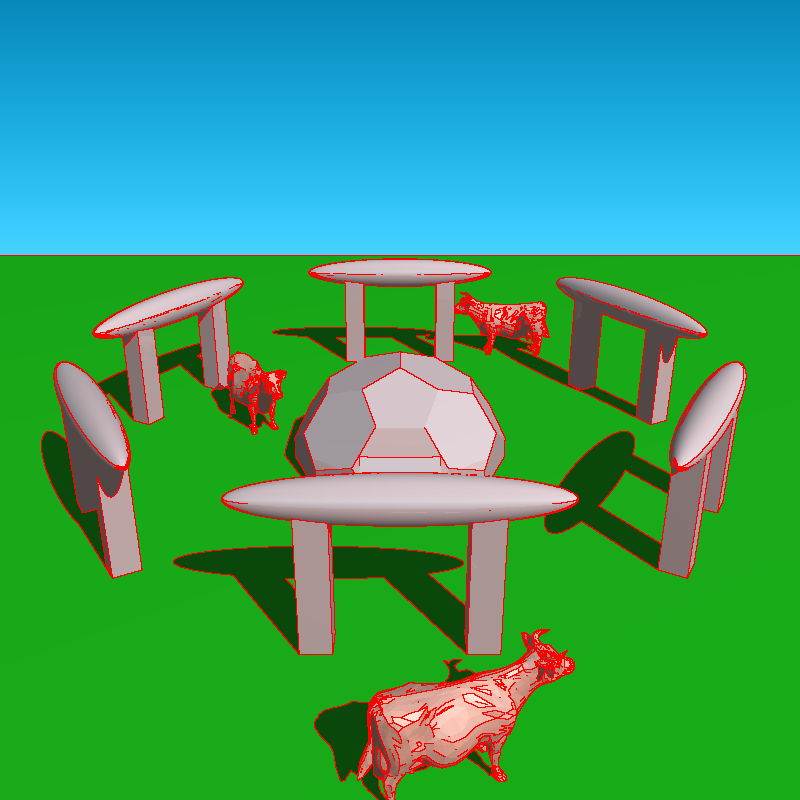
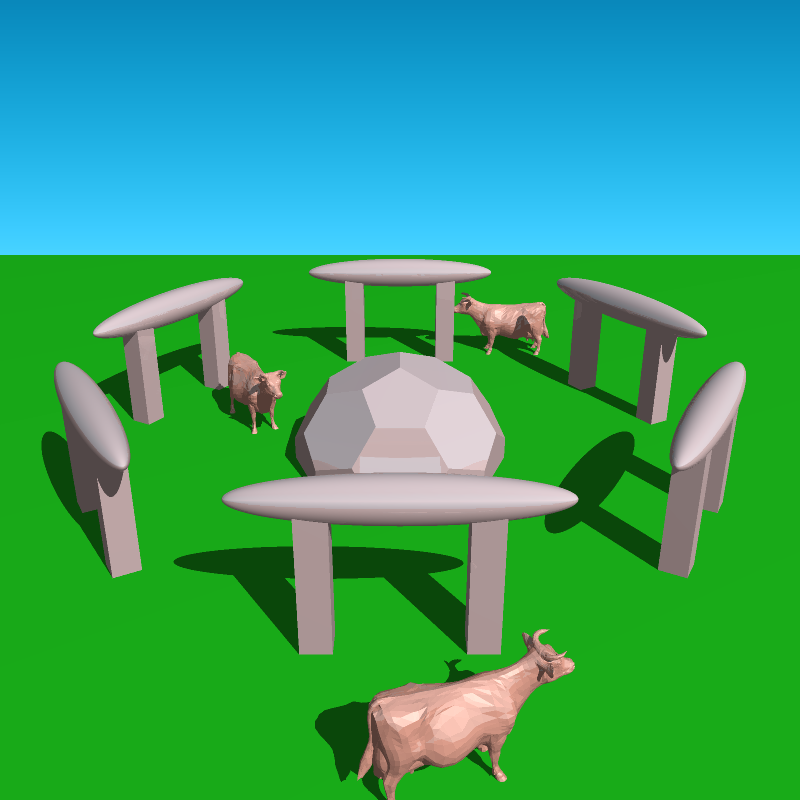
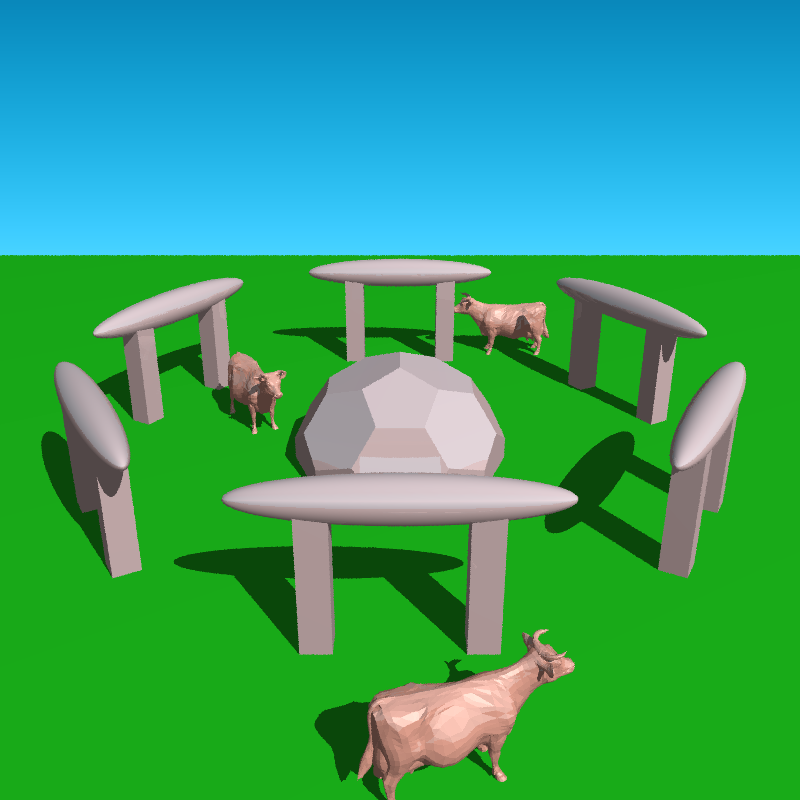
Objective 8: Additional Primitives
See Objectives 3 and 4 for examples of implemented primitives.
Objective 9: Constructive Solid Geometry
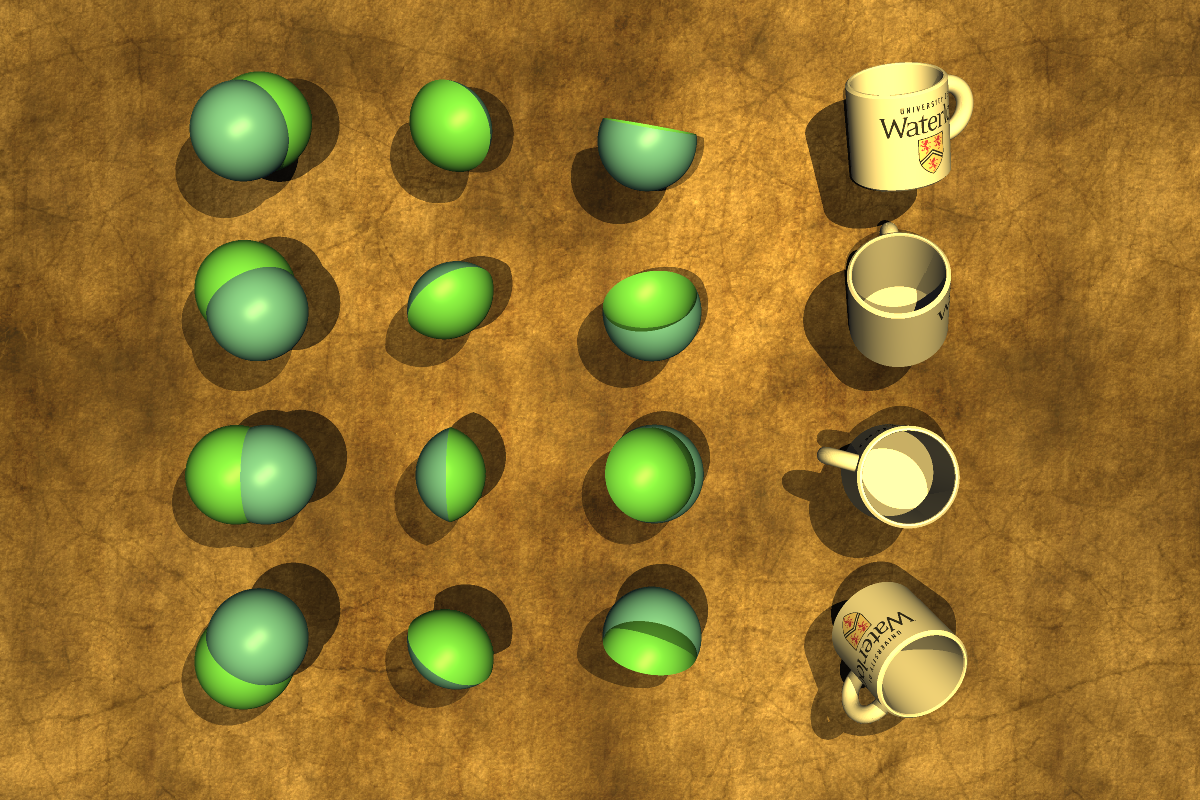
Objective 10: Rube Goldberg machine
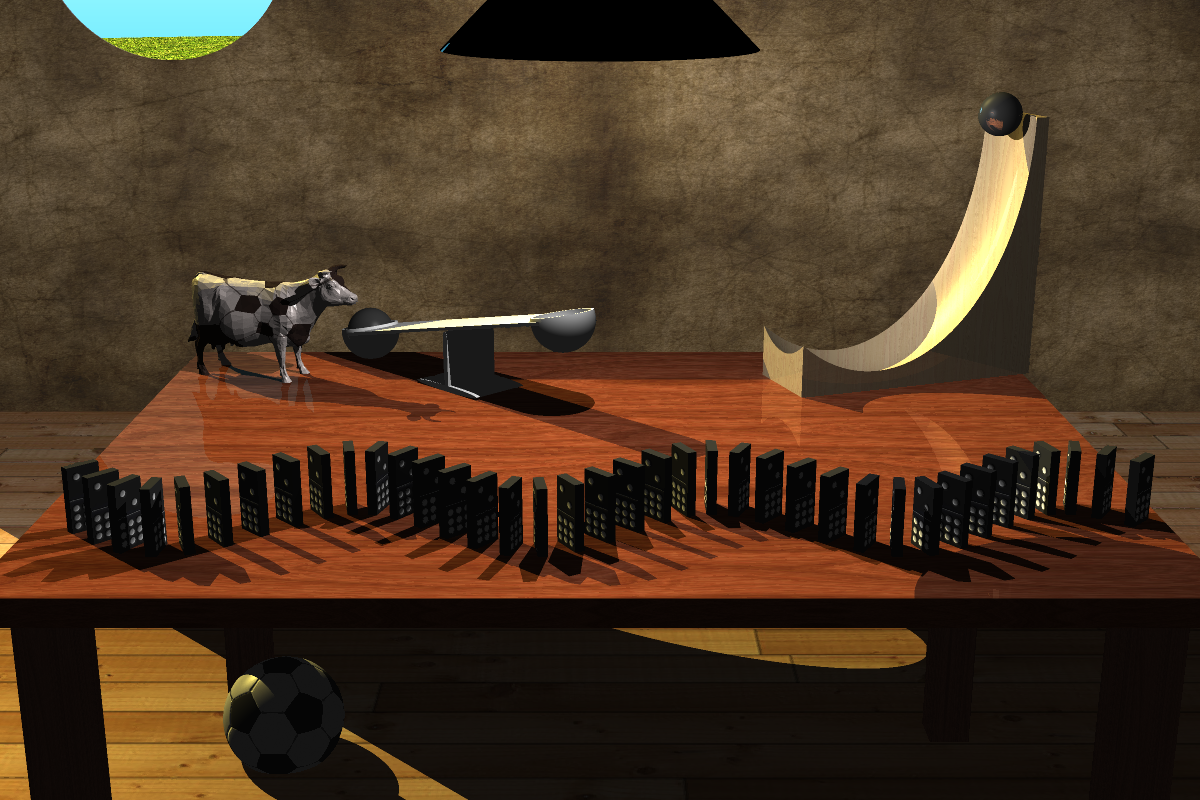